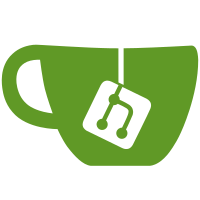
Lors d'une sauvegarde d'une configuration de points, que les points non affichés pour cette prévisualisation ne sont pas conservés.
125 lines
3.4 KiB
C
125 lines
3.4 KiB
C
#include "suppression.h"
|
|
|
|
ErrEnum supprimePoints(const Point tabPoint[],uint32_t nbPoints, const Agglomerat tabAgglo[],uint32_t nbAgglos, const char *const dest, const Mode mode)
|
|
{
|
|
FILE * fdest=fopen(dest,"w");
|
|
if (fdest==NULL)
|
|
{
|
|
fprintf(stderr,"Suppression --> Ouverture destination: %s\n",strerror(errno));
|
|
return MISSING_FILE;
|
|
}
|
|
|
|
char line[SIZE];
|
|
char tmp[SIZE];
|
|
uint32_t prochainAgASupprimer=0;
|
|
for(prochainAgASupprimer = 0; prochainAgASupprimer < nbAgglos; prochainAgASupprimer++)
|
|
{
|
|
if(tabAgglo[prochainAgASupprimer].aSupprimer == true)
|
|
break;
|
|
}
|
|
|
|
for(uint32_t i=0; i<nbPoints;i++)
|
|
{
|
|
/*Tant que le point actuel est un début d'agglomérat à supprimer on saute cet
|
|
* agglomérat*/
|
|
while(prochainAgASupprimer < nbAgglos && tabAgglo[prochainAgASupprimer].agglo[0] - tabPoint == i)
|
|
{
|
|
i+=tabAgglo[prochainAgASupprimer].nbPts;
|
|
prochainAgASupprimer++;
|
|
for(; prochainAgASupprimer < nbAgglos; prochainAgASupprimer++)
|
|
{
|
|
if(tabAgglo[prochainAgASupprimer].aSupprimer == true)
|
|
break;
|
|
}
|
|
}
|
|
|
|
if(i>=nbPoints) /*Gère le cas où on termine sur un agglomérat à supprimer*/
|
|
{
|
|
break;
|
|
}
|
|
|
|
Route* r = (Route*)tabPoint[i].ptOnStructure;
|
|
|
|
if(tabPoint[i].aSupprimer == false && !(mode == MODE_PREVIEW_SUGG &&
|
|
tabPoint[i].type == ROUTE &&
|
|
((r->ptAgglo[0] != NULL && r->ptAgglo[0]->aSupprimer == true) ||
|
|
(r->ptAgglo[1] != NULL && r->ptAgglo[1]->aSupprimer == true))))
|
|
{
|
|
*line='\0';
|
|
strcat(line,"date:");
|
|
sprintf(tmp,"%ld",tabPoint[i].date);
|
|
strcat(line,tmp);
|
|
strcat(line,",lat:");
|
|
sprintf(tmp,"%lf",tabPoint[i].pos.lat);
|
|
strcat(line,tmp);
|
|
strcat(line,",long:");
|
|
sprintf(tmp,"%lf",tabPoint[i].pos.lon);
|
|
strcat(line,tmp);
|
|
strcat(line,";\n");
|
|
fputs(line,fdest);
|
|
}
|
|
}
|
|
|
|
if(fclose(fdest) == EOF)
|
|
{
|
|
fprintf(stderr, "Suppression --> Fermeture destination: %s\n", strerror(errno));
|
|
}
|
|
|
|
return SUCCESS;
|
|
}
|
|
|
|
|
|
void setASupprimerPoint(Point * pt, bool aSupprimer)
|
|
{
|
|
pt->aSupprimer = aSupprimer;
|
|
}
|
|
|
|
void setASupprimerAgglo(Agglomerat * ag, bool aSupprimer)
|
|
{
|
|
ag->aSupprimer = aSupprimer;
|
|
}
|
|
|
|
void setASupprimerPointsInCercle(Cercle cercle, sfCircleShape ** tabPointeursCercles, uint32_t nbPointsAffiches, Point ** ptsAffiches, bool aSupprimer)
|
|
{
|
|
for(uint32_t i=0;i < nbPointsAffiches;i++)
|
|
{
|
|
if(mouseIsInCircle(sfCircleShape_getPosition(tabPointeursCercles[i]), cercle))
|
|
{
|
|
setASupprimerPoint(ptsAffiches[i], aSupprimer);
|
|
}
|
|
}
|
|
}
|
|
|
|
void setASupprimerAgglosInCercle(Cercle cercle, sfCircleShape ** tabPointeursCercleAgglos, Agglomerat * agglos, uint32_t nbAgglos, bool aSupprimer)
|
|
{
|
|
for(uint32_t i=0;i < nbAgglos;i++)
|
|
{
|
|
if(mouseIsInCircle(sfCircleShape_getPosition(tabPointeursCercleAgglos[i]), cercle))
|
|
{
|
|
setASupprimerAgglo(agglos + i, aSupprimer);
|
|
}
|
|
}
|
|
}
|
|
|
|
void setASupprimerPointsInRec(Rectangle rec, sfCircleShape ** tabPointeursCercles, uint32_t nbPointsAffiches, Point ** ptsAffiches, bool aSupprimer)
|
|
{
|
|
for(uint32_t i=0;i < nbPointsAffiches;i++)
|
|
{
|
|
if(mouseIsInRect(sfCircleShape_getPosition(tabPointeursCercles[i]),rec))
|
|
{
|
|
setASupprimerPoint(ptsAffiches[i], aSupprimer);
|
|
}
|
|
}
|
|
}
|
|
|
|
void setASupprimerAgglosInRec(Rectangle rec, sfCircleShape ** tabPointeursCercleAgglos, Agglomerat * agglos, uint32_t nbAgglos, bool aSupprimer)
|
|
{
|
|
for(uint32_t i=0;i < nbAgglos;i++)
|
|
{
|
|
if(mouseIsInRect(sfCircleShape_getPosition(tabPointeursCercleAgglos[i]), rec))
|
|
{
|
|
setASupprimerAgglo(agglos + i, aSupprimer);
|
|
}
|
|
}
|
|
}
|