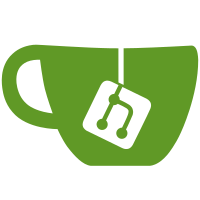
L'utilisateur peut maintenant sélectionner une route entière à supprimer en cliquant sur un point de la route en ayant le bouton alt enfoncé.
93 lines
3.1 KiB
C
93 lines
3.1 KiB
C
#include "controleSuppression.h"
|
|
|
|
|
|
void controleSelectionSuppression(sfRenderWindow * const window, sfEvent * const event, sfSprite * renderSprite, Point ** ptsAffiches, uint32_t nbPointsAffiches, uint32_t pointAffiche, Agglomerat * agglos, uint32_t nbAgglos, uint32_t aggloSelectionne, sfCircleShape ** tabPointeursCercles, sfCircleShape ** tabPointeursCerclesAgglos, const bool aSupprimer)
|
|
{
|
|
if(sfKeyboard_isKeyPressed(sfKeyLControl))
|
|
{
|
|
Rectangle recTrace = selectionRectangle(window, event, renderSprite, aSupprimer);
|
|
/*Si l'utilisateur a juste cliqué, on sélectionne que le point en dessous du
|
|
* curseur s'il y en a un*/
|
|
if(recTrace.positionHG.x == recTrace.positionBD.x && recTrace.positionHG.y == recTrace.positionBD.y)
|
|
{
|
|
if(pointAffiche != nbPointsAffiches)
|
|
{
|
|
if(ptsAffiches[pointAffiche]->type == ROUTE && sfKeyboard_isKeyPressed(sfKeyLAlt))
|
|
{
|
|
Route * r = (Route *)ptsAffiches[pointAffiche]->ptOnStructure;
|
|
for(uint32_t i=0;i < r->nbPts;i++)
|
|
{
|
|
r->chemin[i]->aSupprimer = aSupprimer;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
setASupprimerPoint(ptsAffiches[pointAffiche], aSupprimer);
|
|
}
|
|
}
|
|
|
|
if(aggloSelectionne != nbAgglos)
|
|
setASupprimerAgglo(agglos + aggloSelectionne, aSupprimer);
|
|
}
|
|
else
|
|
{
|
|
setASupprimerPointsInRec(recTrace,tabPointeursCercles, nbPointsAffiches, ptsAffiches, aSupprimer);
|
|
setASupprimerAgglosInRec(recTrace, tabPointeursCerclesAgglos, agglos, nbAgglos, aSupprimer);
|
|
}
|
|
}
|
|
else
|
|
{
|
|
Cercle cercleTrace = selectionCercle(window, event, renderSprite);
|
|
/*Si l'utilisateur a juste cliqué, on sélectionne que le point en dessous du
|
|
* curseur s'il y en a un*/
|
|
if(cercleTrace.rayon == 0)
|
|
{
|
|
if(pointAffiche != nbPointsAffiches)
|
|
{
|
|
if(ptsAffiches[pointAffiche]->type == ROUTE && sfKeyboard_isKeyPressed(sfKeyLAlt))
|
|
{
|
|
Route * r = (Route *)ptsAffiches[pointAffiche]->ptOnStructure;
|
|
for(uint32_t i=0;i < r->nbPts;i++)
|
|
{
|
|
r->chemin[i]->aSupprimer = aSupprimer;
|
|
}
|
|
}
|
|
else
|
|
{
|
|
setASupprimerPoint(ptsAffiches[pointAffiche], aSupprimer);
|
|
}
|
|
}
|
|
|
|
if(aggloSelectionne != nbAgglos)
|
|
setASupprimerAgglo(agglos + aggloSelectionne, aSupprimer);
|
|
}
|
|
else
|
|
{
|
|
setASupprimerPointsInCercle(cercleTrace, tabPointeursCercles, nbPointsAffiches, ptsAffiches, aSupprimer);
|
|
setASupprimerAgglosInCercle(cercleTrace, tabPointeursCerclesAgglos, agglos, nbAgglos, aSupprimer);
|
|
}
|
|
}
|
|
}
|
|
|
|
|
|
void suppressionAndVerbosity(sfRenderWindow *const window, const sfSprite *const renderSprite, const Point tabPoint[], uint32_t nbPoints, const Agglomerat tabAgglo[], uint32_t nbAgglos, const Mode mode)
|
|
{
|
|
char pathSecuredFile[MAXPATHLENGTH];
|
|
|
|
const char *temp = chooseSavingFile(window, renderSprite);
|
|
if(temp == NULL)
|
|
{
|
|
return;
|
|
}
|
|
|
|
strncpy(pathSecuredFile, temp, MAXPATHLENGTH);
|
|
|
|
supprimePoints(tabPoint,nbPoints, tabAgglo, nbAgglos, pathSecuredFile, mode);
|
|
|
|
char string[MAXPATHLENGTH] = "Des logs sans les points supprimés ont été générés dans \'";
|
|
strncat(string, pathSecuredFile, MAXPATHLENGTH - strlen(string));
|
|
strncat(string, "\'.", MAXPATHLENGTH - strlen(string));
|
|
|
|
messageBox(window, renderSprite, string);
|
|
}
|