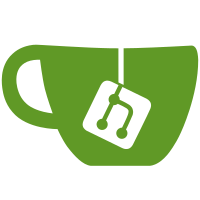
Change la couleur par défaut du soft' Optimisations (mémoire + opérations graphiques menu démarrage) Fix' d'un glitch
94 lines
2.1 KiB
C
94 lines
2.1 KiB
C
#include "deplacement.h"
|
|
|
|
|
|
void scrollUpDisplay(sfRenderWindow *const window, sfSprite *const sprite)
|
|
{
|
|
sfVector2f positionTemp;
|
|
|
|
const double ratio = 5.f;
|
|
const uint16_t endLoop = sfRenderWindow_getSize(window).y / ratio;
|
|
|
|
sfRenderWindow_setFramerateLimit(window, FRAMERATELIMITPOWER);
|
|
|
|
for(uint16_t i = 0; i < endLoop; i++)
|
|
{
|
|
positionTemp = sfSprite_getPosition(sprite);
|
|
sfSprite_setPosition(sprite, (sfVector2f){positionTemp.x, positionTemp.y - ratio});
|
|
|
|
displayBackgroundSprite(window, sprite);
|
|
|
|
// Pour que l'utilisateur puisse fermer la fenêtre, même pendant le scroll' !
|
|
sfEvent event;
|
|
while(sfRenderWindow_pollEvent(window, &event))
|
|
{
|
|
if(event.type == sfEvtClosed || sfKeyboard_isKeyPressed(sfKeyEscape))
|
|
{
|
|
exit(EXIT_SUCCESS);
|
|
}
|
|
}
|
|
}
|
|
|
|
sfRenderWindow_setFramerateLimit(window, FRAMERATELIMITSLEEP);
|
|
}
|
|
|
|
|
|
bool deplacerSpriteTouchesDirectionnelles(sfSprite *const sprite)
|
|
{
|
|
static int8_t offset_x, offset_y;
|
|
static bool flag;
|
|
|
|
offset_x = 0;
|
|
offset_y = 0;
|
|
flag = false;
|
|
|
|
if(sfKeyboard_isKeyPressed(sfKeyLeft) || sfKeyboard_isKeyPressed(sfKeyNumpad4))
|
|
{
|
|
flag = true;
|
|
|
|
if(sfSprite_getPosition(sprite).x - WIDTH * (OFFSETFORMOTION / 100.0) >= 0)
|
|
{
|
|
offset_x = -OFFSETFORMOTION;
|
|
}
|
|
}
|
|
|
|
if(sfKeyboard_isKeyPressed(sfKeyRight) || sfKeyboard_isKeyPressed(sfKeyNumpad6))
|
|
{
|
|
flag = true;
|
|
|
|
if(sfSprite_getPosition(sprite).x + MAPSIZE * MAPSCALE + WIDTH * (OFFSETFORMOTION / 100.0) < WIDTH)
|
|
{
|
|
offset_x = OFFSETFORMOTION;
|
|
}
|
|
}
|
|
|
|
if(sfKeyboard_isKeyPressed(sfKeyUp) || sfKeyboard_isKeyPressed(sfKeyNumpad8))
|
|
{
|
|
flag = true;
|
|
|
|
if(sfSprite_getPosition(sprite).x - HEIGHT * (OFFSETFORMOTION / 100.0) >= 0)
|
|
{
|
|
offset_y = -OFFSETFORMOTION;
|
|
}
|
|
}
|
|
|
|
if(sfKeyboard_isKeyPressed(sfKeyDown) || sfKeyboard_isKeyPressed(sfKeyNumpad2))
|
|
{
|
|
flag = true;
|
|
|
|
if(sfSprite_getPosition(sprite).x + MAPSIZE * MAPSCALE + HEIGHT * (OFFSETFORMOTION / 100.0) < HEIGHT)
|
|
{
|
|
offset_y = OFFSETFORMOTION;
|
|
}
|
|
}
|
|
|
|
if(flag)
|
|
{
|
|
offset_x *= WIDTH / 100.0;
|
|
offset_y *= HEIGHT / 100.0;
|
|
|
|
sfSprite_move(sprite, (sfVector2f){offset_x, offset_y});
|
|
}
|
|
|
|
return flag;
|
|
}
|