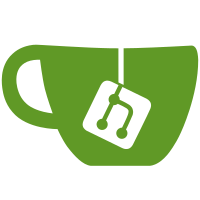
Intègre le basculement des modes PBP vers le GLOBAL Diminue le nombre d'icônes max' du menu
127 lines
2.6 KiB
C
127 lines
2.6 KiB
C
#pragma once
|
|
|
|
#include <inttypes.h>
|
|
#include <stdbool.h>
|
|
#include <time.h>
|
|
#include <SFML/Graphics.h>
|
|
|
|
#include "constantes.h"
|
|
|
|
|
|
typedef struct Agglomerat Agglomerat;
|
|
typedef struct Route Route;
|
|
typedef struct AgglomeratGlobal AgglomeratGlobal;
|
|
|
|
typedef enum {NONE, AGGLOMERAT, ROUTE} TypePt;
|
|
|
|
typedef enum {NO_MODE, MODE_GLOBAL, MODE_PBP_AUTOMATIC, MODE_PBP_MANUAL, MODE_PREVIEW, MODE_PREVIEW_SUGG, MODE_MULTI_FILES} Mode;
|
|
|
|
typedef enum {NO_ACTION, ACTION_QUIT, ACTION_ZOOM_NEXT, ACTION_ZOOM_PREV, ACTION_ZOOM_ZONE, ACTION_CHANGE_MAP_TYPE, ACTION_ZOOM_IN, ACTION_ZOOM_OUT, ACTION_ZOOMABLE, ACTION_UNZOOMABLE, ACTION_CENTERPOSITION, ACTION_CHANGE_VISUALISATION, ACTION_REFRESH, ACTION_REPEAT, ACTION_TOGGLEAGGLO, ACTION_TOGGLEROUTE} Action;
|
|
|
|
typedef enum {FIRSTYPE, ROADMAP, SATELLITE, TERRAIN, HYBRID, LASTYPE} MapType;
|
|
|
|
typedef enum {NO_TOOL, TOOL_EXIT, TOOL_BACKTOHOME, TOOL_ZOOMZONE, TOOL_SELECTPOINTS, TOOL_DESELECTPOINTS, TOOL_ADDLOGS, TOOL_ACCEPT, TOOL_GLOBAL, TOOL_NEXT, TOOL_PREVIOUS, TOOL_MODE_PBP, TOOL_MODE_GLOBAL, TOOL_RESUME, TOOL_PAUSE, TOOL_CONTINUE, TOOL_CENTERPOSITION, TOOL_REPEAT, TOOL_TOGGLEAGGLO, TOOL_TOGGLEROUTE, TOOL_CHANGEMAP, TOOL_ZOOMPREV, TOOL_ZOOMNEXT, TOOL_PREVIEW, TOOL_SAVE, TOOL_ERASE} Tool;
|
|
|
|
typedef struct
|
|
{
|
|
double lat;
|
|
double lon;
|
|
} Coordonnees;
|
|
|
|
typedef struct
|
|
{
|
|
time_t date;
|
|
Coordonnees pos;
|
|
sfVector2f posConv;
|
|
TypePt type;
|
|
void *ptOnStructure;
|
|
bool aSupprimer;
|
|
} Point;
|
|
|
|
struct Agglomerat
|
|
{
|
|
Coordonnees moy;
|
|
sfVector2f moyConv;
|
|
Point **agglo;
|
|
uint32_t nbPts;
|
|
Route *ptRoute[2];
|
|
double rayon;
|
|
double tailleCercle;
|
|
bool aSupprimer;
|
|
};
|
|
|
|
struct AgglomeratGlobal
|
|
{
|
|
Coordonnees moy;
|
|
sfVector2f moyConv;
|
|
Agglomerat **assemblage;
|
|
uint32_t nbAgglo;
|
|
uint32_t nbPtsGlobal;
|
|
double tailleCercle;
|
|
char *adresse;
|
|
};
|
|
|
|
struct Route
|
|
{
|
|
Point **chemin;
|
|
uint32_t nbPts;
|
|
Agglomerat *ptAgglo[2];
|
|
};
|
|
|
|
typedef enum
|
|
{
|
|
SUCCESS,
|
|
LOAD_SUCCESS,
|
|
MISSING_FILE,
|
|
CORRUPTED_FILE,
|
|
ALLOCATION_FAILURE,
|
|
REQUEST_FAILURE,
|
|
MODE_FAILURE
|
|
} ErrEnum;
|
|
|
|
typedef struct
|
|
{
|
|
sfVector2f centre;
|
|
double rayon;
|
|
} Cercle;
|
|
|
|
typedef struct
|
|
{
|
|
sfVector2f positionHG;
|
|
sfVector2f positionBD;
|
|
} Rectangle;
|
|
|
|
typedef struct
|
|
{
|
|
uint8_t zoom;
|
|
MapType type;
|
|
double echelle;
|
|
sfSprite *mapSprite;
|
|
sfTexture *mapTexture;
|
|
Coordonnees pointCentral;
|
|
Coordonnees pointHautGauche;
|
|
Coordonnees pointBasDroite;
|
|
} Carte;
|
|
|
|
typedef struct
|
|
{
|
|
Carte *pile[MAPSTACKSIZE];
|
|
int16_t sommet;
|
|
} PileCartes;
|
|
|
|
typedef struct
|
|
{
|
|
Tool outil;
|
|
Rectangle zone;
|
|
char nom[BUTTONAMESIZE];
|
|
sfSprite *spriteButton;
|
|
sfTexture *textureButton;
|
|
} Bouton;
|
|
|
|
typedef struct
|
|
{
|
|
int16_t sommetTableau;
|
|
Bouton *liste[MAXBUTTONSMENU];
|
|
sfRenderTexture *renderTextureMenu;
|
|
} Menu;
|